JWT Validation
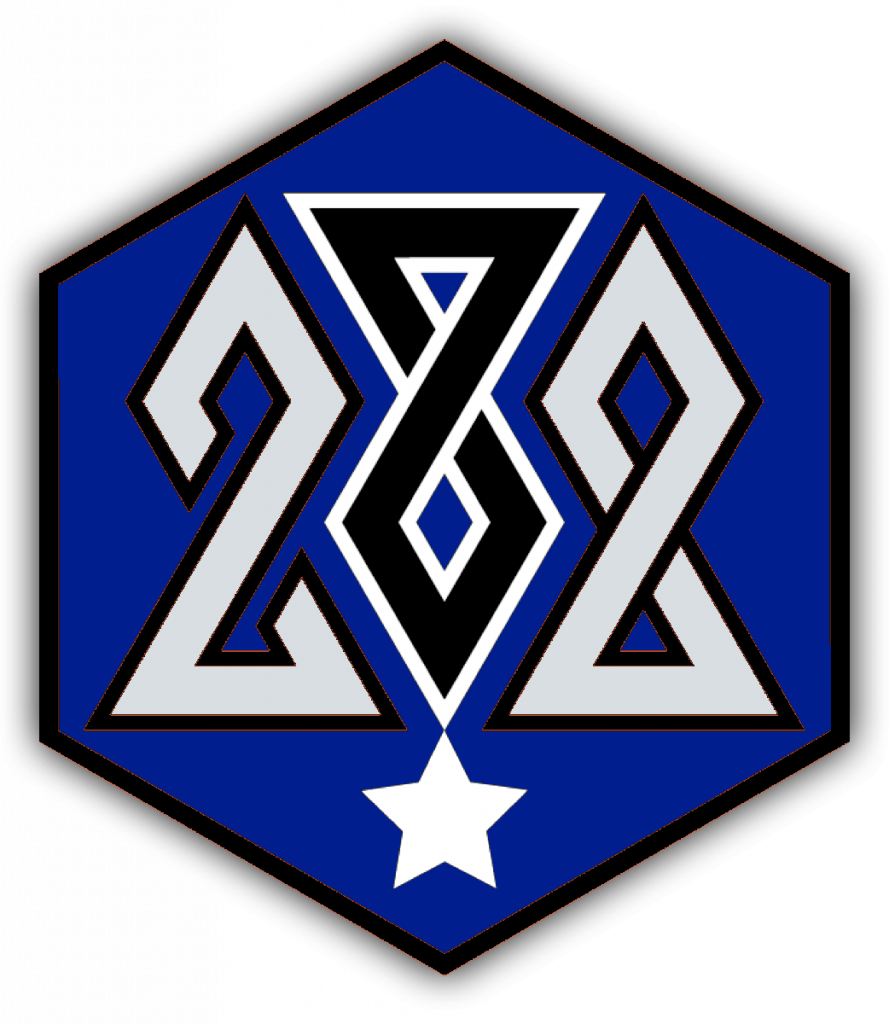
.Net Core
To properly validate JSON Web Tokens (JWTs) in .NET Core and defend against JWT theft and tampering, developers should follow these best practices:
- Use a Secure JWT Library: Utilize a trusted and well-maintained JWT library specifically designed for .NET Core, such as the ones below. These libraries provide robust functionality for JWT validation and help mitigate common security risks.
Microsoft.IdentityModel.Tokens
System.IdentityModel.Tokens.Jwt
Microsoft.AspNetCore.Authentication.JwtBearer
- Validate the Token Signature: Verify the integrity of the JWT by validating its signature. This involves verifying that the token has not been tampered with and was signed by a trusted party. Ensure that the library performs signature validation against the correct signing key or certificate.
- Verify Token Expiration (Exp) and Not Before (Nbf) Claims: Check the “exp” (expiration time) and “nbf” (not before) claims in the JWT to ensure that the token is still valid and within the specified time range. Reject tokens that have expired or are not yet valid to prevent the use of stale or tampered tokens.
- Validate Token Audience (Aud) and Issuer (Iss) Claims: Verify that the “aud” (audience) and “iss” (issuer) claims in the JWT match the expected values. This ensures that the token is intended for the specific audience and issued by a trusted issuer.
- Implement Custom Validation Rules: Implement additional custom validation rules based on your application’s requirements. This may include verifying specific claims, checking user roles or permissions, or applying any business-specific validation logic.
- Securely Store and Protect Keys: Safely store the signing key or certificate used for JWT verification. Use secure storage mechanisms, such as environment variables or Key Vault, to prevent unauthorized access to the keys. Regularly rotate keys and certificates as part of a robust key management strategy.
- Employ HTTPS for Transport Layer Security: Ensure that JWTs are transmitted securely over HTTPS to protect against eavesdropping and MitM attacks. This prevents unauthorized interception or tampering of tokens during transit.
- Implement Defense-in-Depth Measures: Combine JWT validation with other security measures, such as secure session management, strong authentication mechanisms, and input validation, to provide a layered defense against attacks.
- Stay Up-to-Date with Security Patches: Keep your JWT library and dependencies up-to-date with the latest security patches and updates. Regularly review security advisories and apply patches promptly to address any known vulnerabilities.
By following these practices, .NET Core developers can establish a robust JWT validation process, ensuring the integrity and security of their authentication and authorization mechanisms, and defending against JWT theft and tampering.
Bearer Tokens
A bearer token is a type of access token used in authentication and authorization protocols, such as OAuth 2.0. It is called a “bearer” token because the token itself is sufficient to prove the bearer’s identity and authorization rights when making requests to protected resources.
Bearer tokens are typically long, randomly generated strings or tokens issued by an authentication server or an authorization server. Once a client (such as a web or mobile application) obtains a bearer token, it can include the token in the “Authorization” header of subsequent HTTP requests to access protected resources.
Setup an authentication server for issuing bearer tokens in ASP.NET Core using libraries like OpenIddict or IdentityServer4.
Bearer tokens carry the following characteristics:
- Stateless: Bearer tokens are usually stateless, meaning the server doesn’t need to keep track of the token’s state or store it on the server-side. The token contains all the necessary information for authentication and authorization.
- Authorization Grants: Bearer tokens are obtained through an authorization grant process. The client presents a valid authentication grant (such as a username/password or a previously obtained refresh token) to the authorization server, which then issues the bearer token.
- Authorization Scopes: Bearer tokens may contain authorization scopes, which define the specific permissions or access rights the bearer has. These scopes determine what actions the bearer is allowed to perform on protected resources.
- Token Lifespan: Bearer tokens have a limited lifespan or expiration time defined by the issuing authority. Once expired, the client needs to obtain a new token using the refresh token or reauthenticate to continue accessing protected resources.
- Transport Security: Bearer tokens must be transmitted securely to prevent interception or tampering. They are typically sent over HTTPS, providing encryption and integrity protection during transit.
It’s important to note that bearer tokens should be treated as sensitive information. They grant access to protected resources, and if compromised, an attacker can impersonate the bearer. Therefore, it’s crucial to protect bearer tokens from unauthorized access and implement secure token management practices, such as secure storage, token revocation mechanisms, and token expiration policies.