ASP.Net Core Authentication
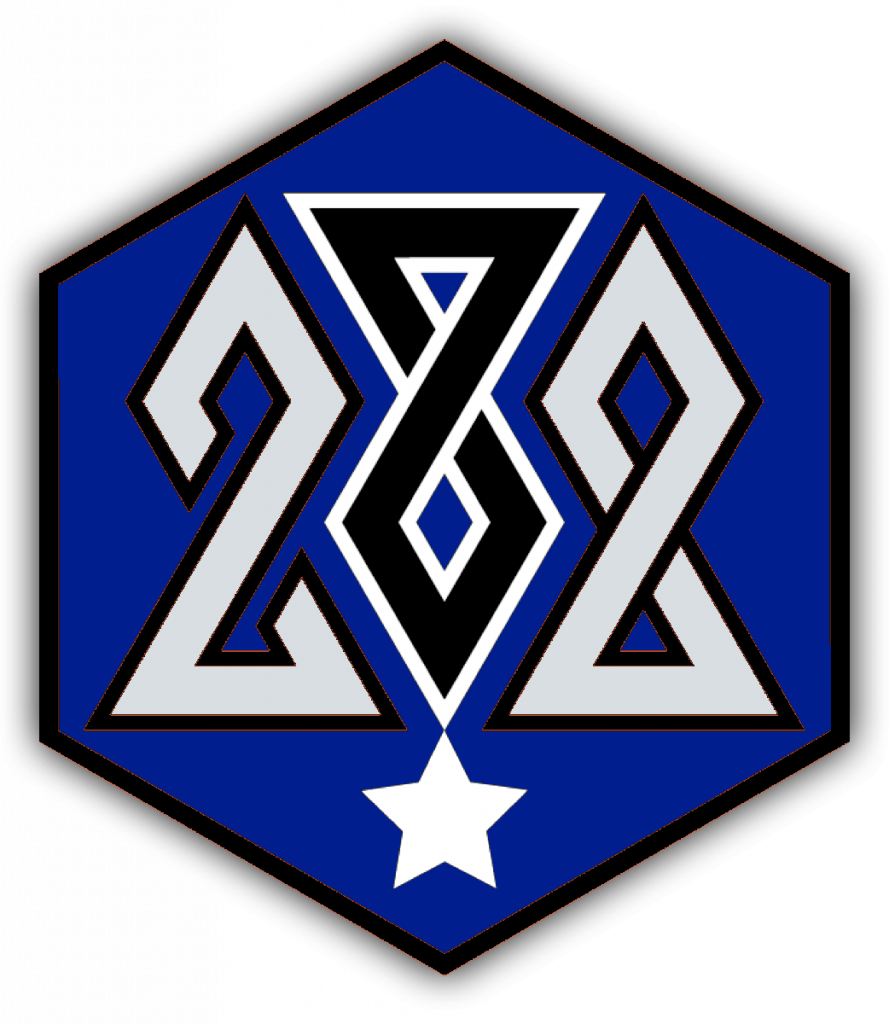
Anyone else tired of researching an advanced topic, but first you have to impatiently wait for or skip over the first 80% of the demo/example/video in which the application is once again created from scratch using the same Microsoft template as every other example? If you are researching an advanced topic that their own title suggests, then it’s implied that you already know the basics. Stop wasting my time with fluff, I’m looking for the advanced part.
In many cases that application that requires me to do this research, was already created by someone else and I inherited it. So how do I piece together how it works? Reverse engineering. Here is an example of how I figured out how ASP.Net Core authentication works and potentially what it’s vulnerable to, if misconfigured.
I usually start with actually using the web application like a normal user would, but with Burp Suite Pro passively scanning and building a site map for me. The application that I’m targeting here is an internal application codenamed ProjectBravo. Browsing to it, take me directly to a login page. The URI is /Identity/Account/Login, which looks MVC-ish to me.
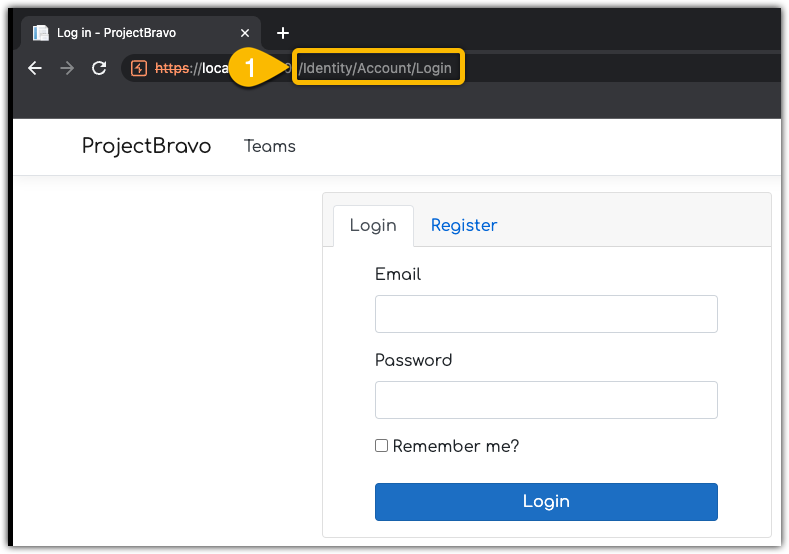
I don’t have a login so, I’ll click Register and create an account. This is looking more like MVC, the URI is now /Identity/Account/Register
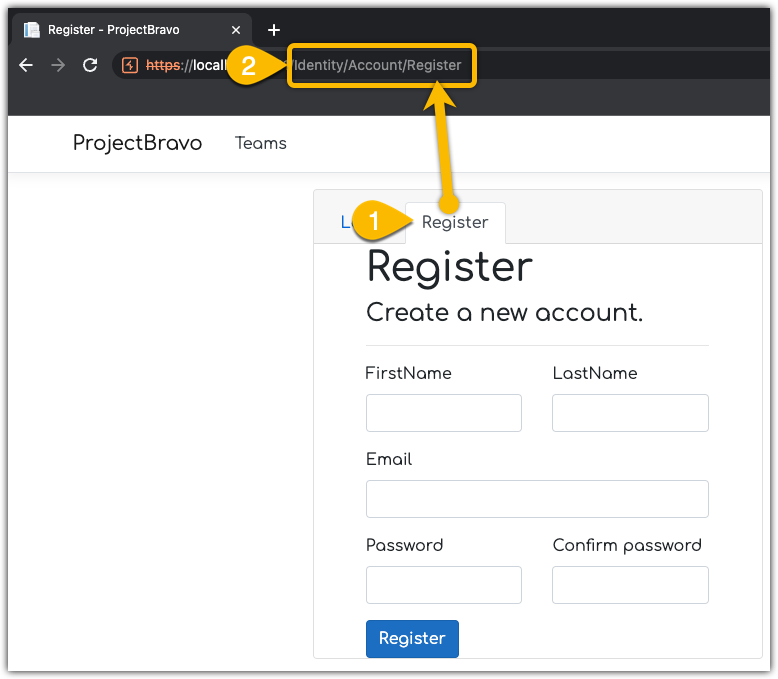
I typical enter values into each box, then delete it or tab to the next one to see what input validation messages are returned (if any). It looks like every field on the page is required. One thing pops out immediately, that is the message is stating that the minimum length of the password is only 6 characters, which is vulnerable to brute forcing in a short amount of time. I hope it’s using all available character sets (uppercase, lowercase, numbers, and a lot of symbols).
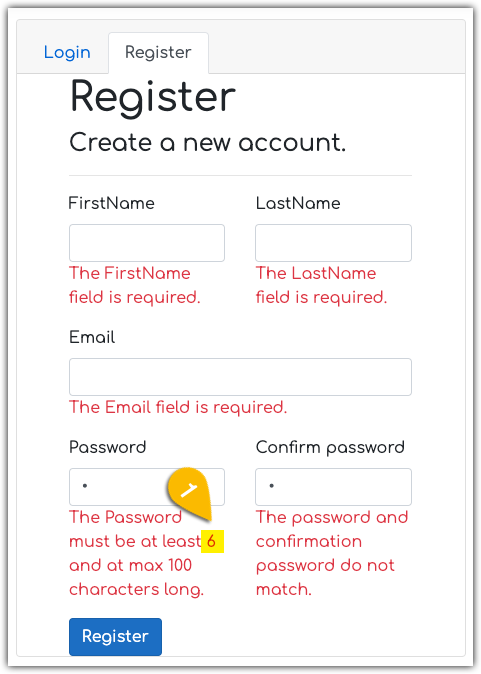
To keep this moving, I’ll provide valid values to create an account. I’ll just use a fake name & email to get past this part.
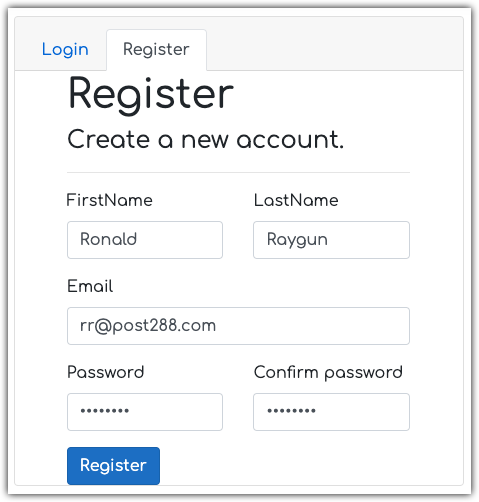
The Request
Before I click Register, I turn Burp Suite’s proxy to intercept. Here is the captured POST.
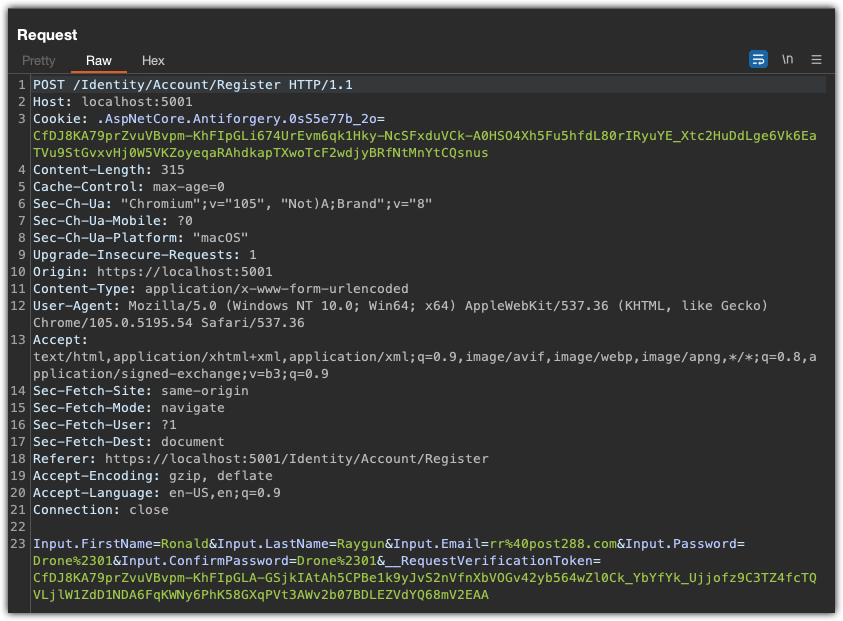

On line 3, I notice this cookie, .AspNetCore.Antiforgery.0sS5e77b_2o=
is being set. A few questions come to mind:
- Where is this coming from?
- What does the random looking part (
.0sS5e77b_2o
) of the cookie name do ? - What is the cookie value used for?
Doing some quick googling takes me here, https://docs.microsoft.com/en-us/aspnet/core/security/anti-request-forgery?view=aspnetcore-6.0 where I get a couple quick answers.
- The cookie is coming from the Microsoft.AspNetCore.Antiforgery Namespace.
- I still don’t know where random suffix of the cookie name (
.0sS5e77b_2o
) is coming from. Hopefully, I will uncover this later in my research. - The intent of the cookie is to prevent Cross-site request forgery (CSRF).
namespace Microsoft.AspNetCore.Antiforgery
Here comes a rabbit hole… While I’m looking at the assemblies, I locate the target namespace, but also find other interesting Authentication namespaces that might come into play later on.
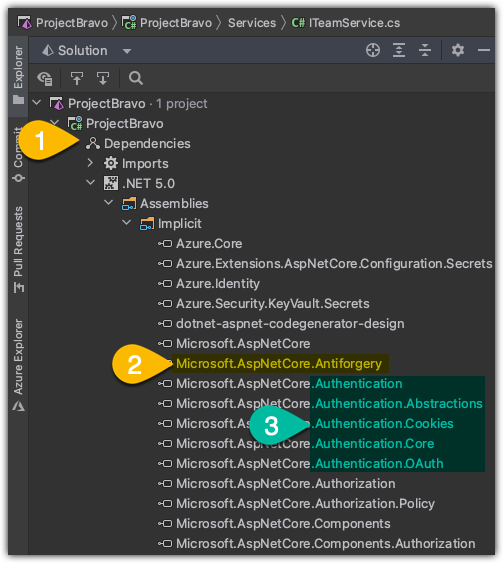
OK, refocus. Where in the code does this come into play? I’m doing a string search AntiForgery
and it appears that all of the controllers are in the results. I peek into one of them.
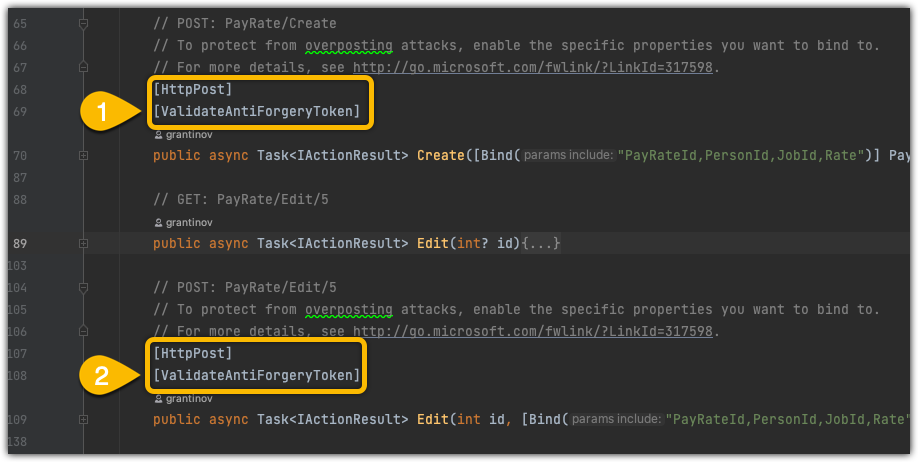
Another question comes to mind. How are attributes wired up in the code? I right click on the [ValidateAntiForgeryToken]
attribute and select Go To Definition. This brings me to the ValidateAntiForgeryTokenAttribute
class in the ValidateAntiForgeryTokenAttribute.cs
file in the Microsoft.AspNetCore.Mvc
namespace. A few more Go To Definition clicks and I quickly realize there is maybe a better way to do this. I’ll just set a breakpoint and debug the process to see how deep the rabbit hole goes.
The Response
And here is the response to the POST
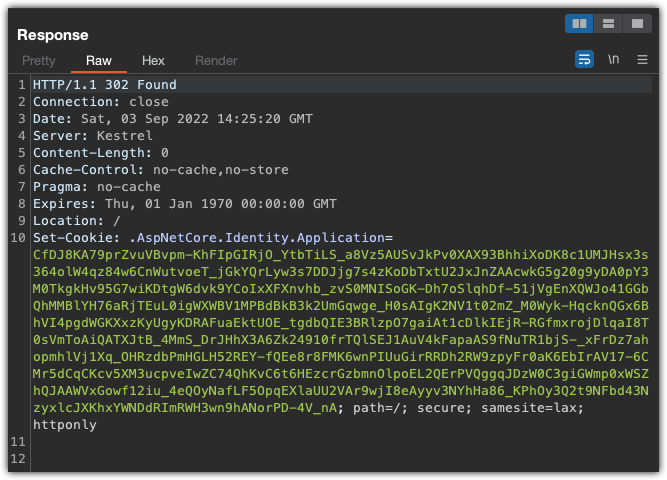
Now another request to GET the page after registering
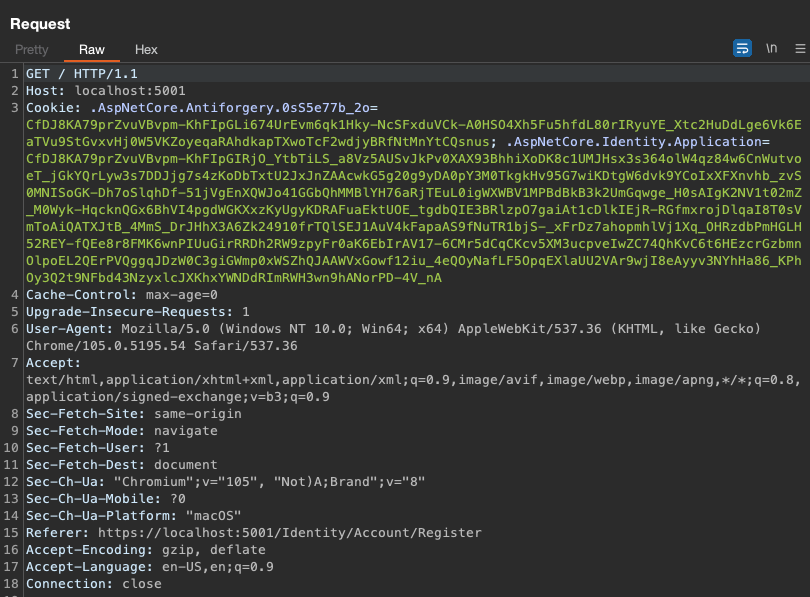
The response to that has a hidden input form field, named __RequestVerificationToken
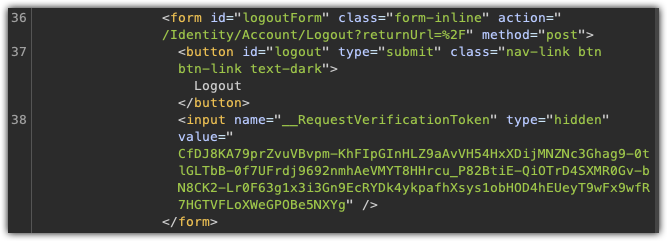
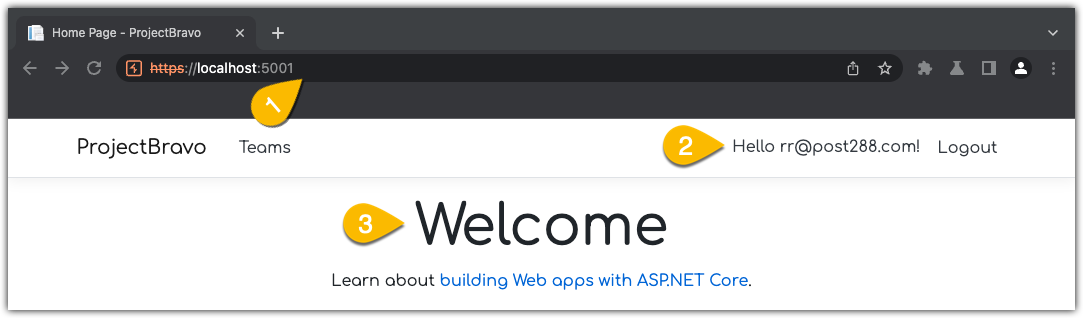
CPE